Programming is a fast-growing industry, and it has become a must-known skill for business people. Python is one of the most popular programming language used in the recent decade. Why is that? Take a look at this article to find out.
Why Programming?
Programming in a Company Context
Information technology is ruling the world. In the early 1990s, companies are leveraging technology to improve their profitability by widening distribution channels, improving employees productivity, and increase sales turnover. Nowadays, however, companies are built on top of technology. For example -
- Amongst the top 10 most valuable companies in 2020, at least 7 of them are technology or Internet-based (Microsoft, Apple, Amazon, Alphabet (Google), Facebook, Alibaba, Tencent)
- UPS utilizes Internet Of Things (IoT) by installing sensors on its trucks. UPS is now a technology company with trucks, instead of a trucking company with technology.
- Companies like Uber and Airbnb are creating a new paradigm of the economy called Sharing Economy.
Challenge: Find a company which doesn’t use information technology. I’ll wait. ⏲
But what EXACTLY is information technology? I can definitely give you the Wikipedia definition of the term. Still, I’d instead try to show you a component view of this term to stress the importance of IT in companies and businesses.
When we say a company uses IT, what we are trying to say is a company builds and uses one or more Information Systems. Each Information System serves a particular business goal for the business and its customer. For example, a hypothetical supermarket, Parkanshop, may have a system for inventory management, another for product checkout, another for online shopping, etc. To build each of these systems, Parkanshop needs to consider at least the following six components:
- Hardware: what devices should we use? Are these devices powerful enough? What if we need to scale up to serve more people?
- Software: how do we let the user interact with the system?
- Network: how do we connect all the components together? Are there security issues?
- Data: how do we store and protect business-critical data?
- Process: what actions will the user and the system take?
- People: who are the users? What do they want to accomplish? How do they feel about the system?
Programming is the action of defining a set of command and instructions for computers to execute. It is one of the most critical skills a company must possess to efficiently deliver business values to their customer.
Programming in a Personal Context
Okay, maybe you don’t own a company. Can programming still help you? Yes. Here is a list of things you may learn to assist your academic study or your work-
- Do you have a business idea? Write a programme to prototype it.
- Are you doing a mundane job with repetitive steps? Automate it.
- Are you sensitive to numbers and statistics? Learn data analysis.
- Are you a creative person? Build an app to express it.
I believe the most valuable benefit of learning to code is - it trains your problem-solving skills. Once you understand how a programmer thinks, you can quickly dissect a problem into different [abstraction] levels. This helps you to solve not just coding problems, but also day-to-day challenges.
Everybody should learn to program a computer, because it teaches you how to think.
Why Python?
According to Wikipedia, our reliable source of knowledge, there are around 700 programming languages in the world. Some of them have fallen out of use, but safely speaking, 50 popular languages are still in use.
So why Python? There are a few reasons.
Python is Popular
According to StackOverflow, the go-to website when we have programming doubt, Python is the 4th most popular and the 3rd most loved programming languages in 2020. Python is renowned for having a supportive society. When you have problems with Python, you will get tremendous support on forums like StackOverflow.
Python is Easy
Python is one of the easiest languages to learn and use. To prove my point, imagine we are writing the most straightforward programme in the world - to display one line on the screen. This is usually called the ‘Hello World’ programme.
In C++, a language famous for its speed and robustness, the ‘Hello World’ programme looks something like this-
#include <iostream>
using namespace std;
int main() {
cout << "Hello World!" << endl;
}
In Java, a language famously used for Android application development, the ‘Hello World’ programme looks like this-
public class HelloWorld {
public static void main(String []args) {
System.out.println("Hello World!");
}
}
Both look complicated, right? How about Python? This is how simple Python is-
print("Hello World!")
Python is Interactive
In other languages, you typically need to write the whole programme before testing in a process called compiling. Compiling means converting the code you type into an executable file which you can double click to run it. However, Python does not require compiling, so you can test each line as you write them. Mostly.
Whom is this Series For?
I believe everyone can pick up the skill of programming. When I plan for writing this series, I try to explain most concepts in a way teenagers would understand.
However, in this series, I’ll focus on, not only the basic Python syntaxes and problem-solving skills, but also how to apply Python in a business context. In the later parts of the series, we will learn about how to use Python for fundamental data analysis, data visualization, and some “hacker statistics”.
I am a BBA(IS) graduate of HKUST. I have personally taken 2 Python programming courses, and I have been a student assistant in teaching Python. My writing will, therefore, resonate with ISOM 2020 or ISOM 3400. So, if you are, or plan to be, a student of these two courses, this may serve as a practical guide for your study. This series, however, is not a replacement for these courses; this is by no means an academic paper, nor is it aligned with any syllabus. My goal with this series is to outline your path to learning programming, not to help you get A grades in a particular course. While I’m happy to tutor anyone privately, please do not expect these articles to be course-complete.
So, if you are
- a business student who is interested in technology or programming, or;
- a business person who wants to add programming into their skill set,
this series is for you.
Installation
I’ll be using the Anaconda distribution of Python. The Anaconda distribution is one of the best distributions for data analyst and scientist because it saves a lot of time on managing a lot of libraries. These libraries are Python files which provide additional functionalities to Python with fewer lines of code. Unless you plan to be a Python developer, you don’t want to manage these libraries yourselves.
Also, Anaconda provides two software called ”Jupyter Notebook” and ”JupyterLab”. This two software is handy for beginners to learn Python because of their easy to use interface.
To download Anaconda, go to the Official Website and click the download button. Select the 64-bit graphical installer.
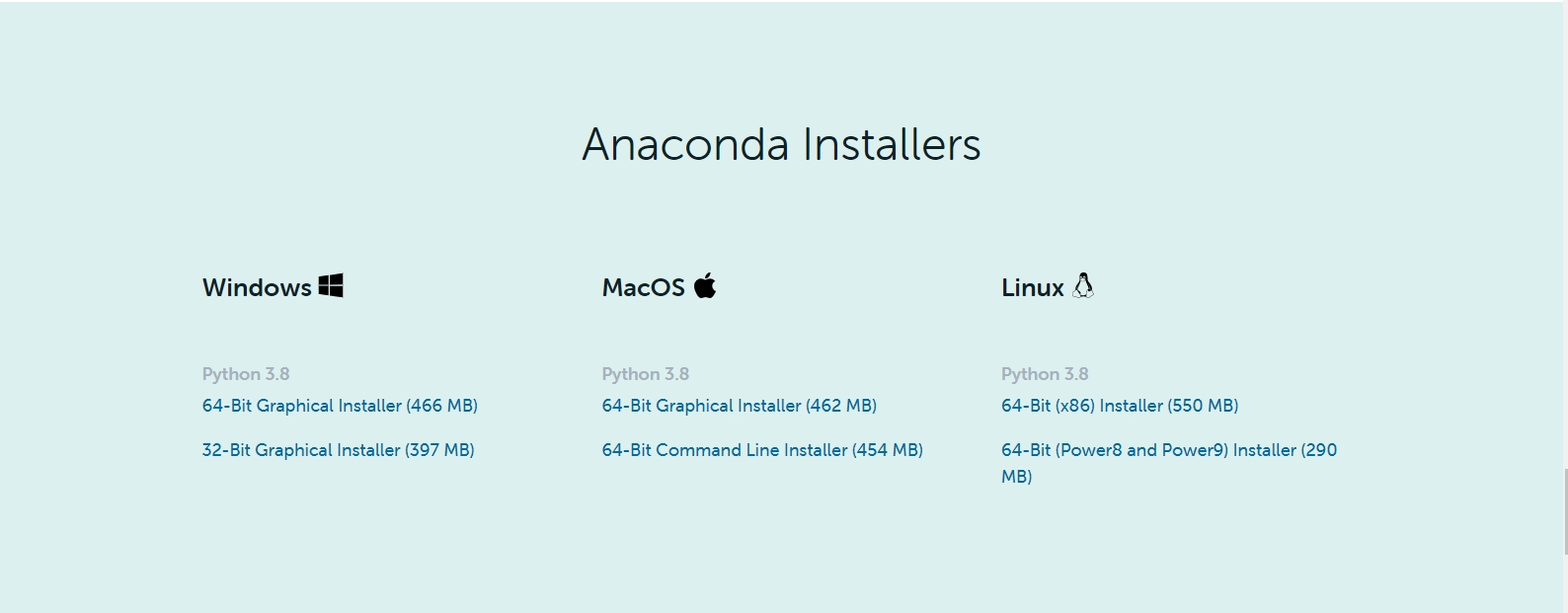
The download page of Anaconda 3 Individual Edition. Select the 64-bit graphical installer option for your operating system.
Afterwards, run the installer. The default settings will suffice in most cases, so patiently press ‘next’ multiple times.
When you finish installing Anaconda, launch “Anaconda Navigator (Anaconda3)” from your start menu or your launchpad. For my writing, I’ll use “JupyterLab” because (1) it’s newer, and I like new stuff, and (2) the user interface is a bit cleaner. Feel free to use “Jupyter Notebook” - they have the same capability. Click the “launch” button to fire your programme.
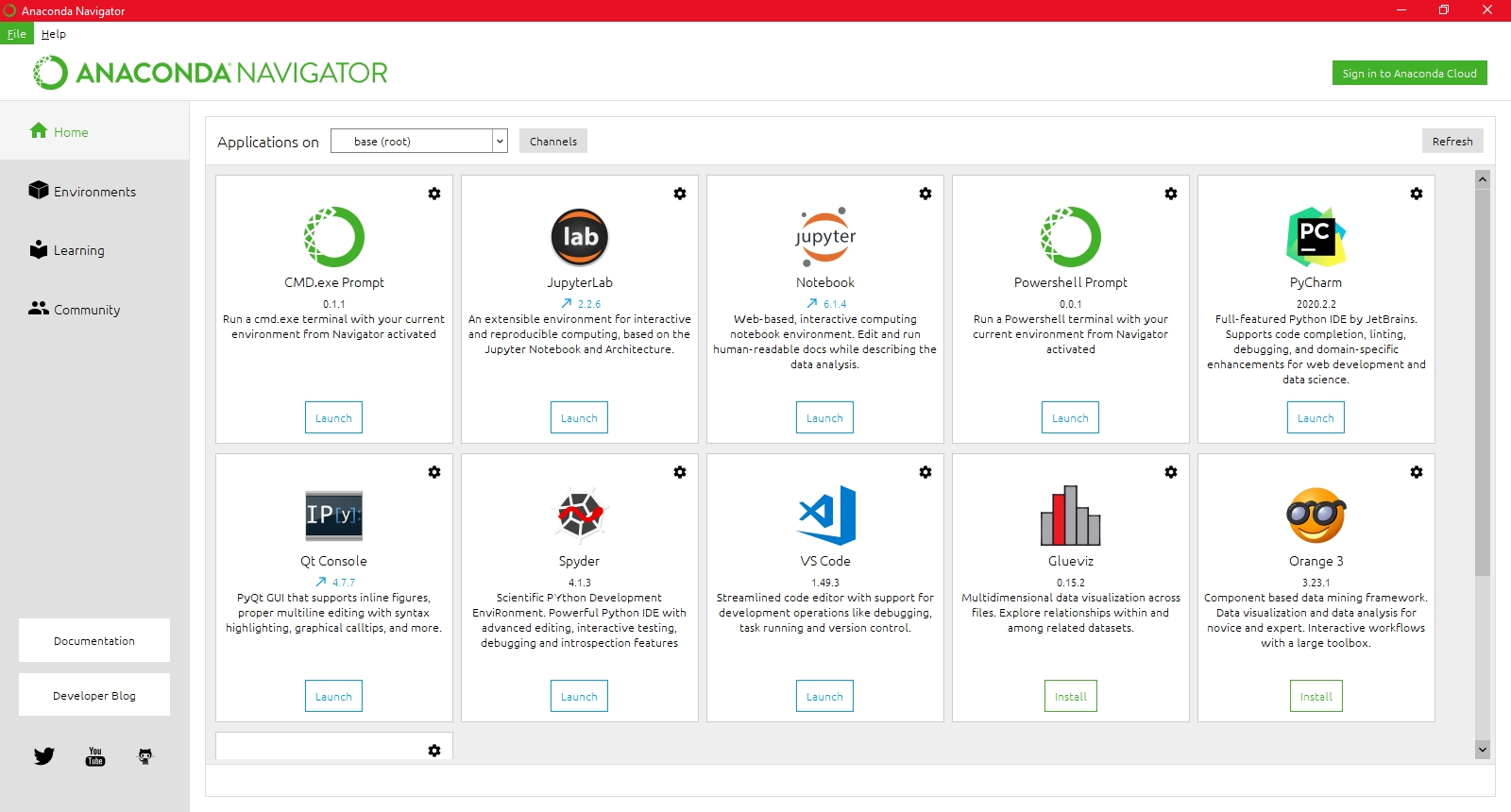
The interface of Anaconda Navigator
A browser will then pop up with JupyterLab. The left side is a navigation bar, which should be showing your user folder on your computer. Create a new folder by pressing the “folder plus” icon, and name the folder “python” (or anything you like). Double click on the new folder, and start a new Python Notebook.
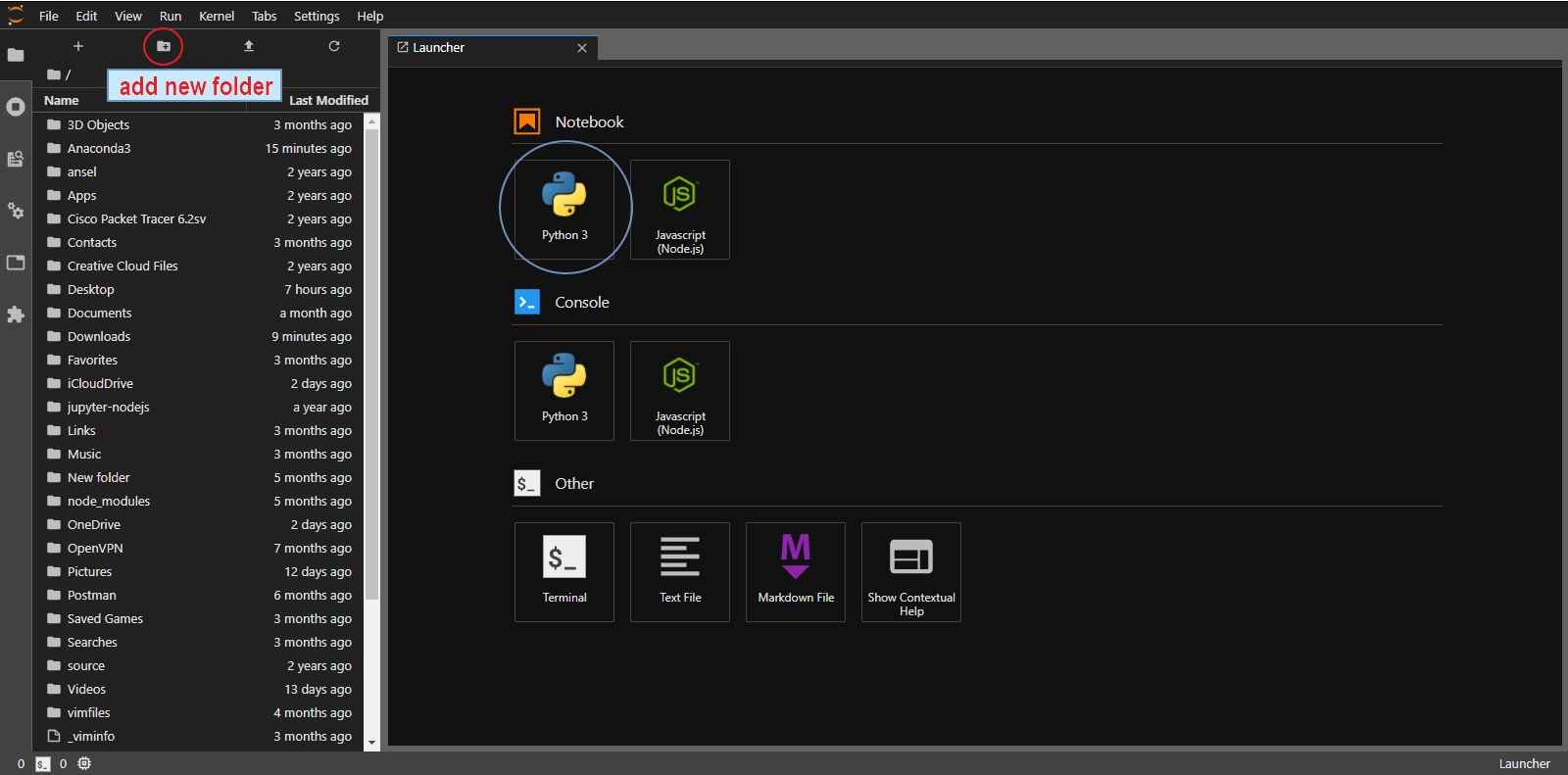
The interface of JupyterLab. I’ve changed to a dark theme because I’m a vampire.
Create a new folder for better file organization, start a new Python notebook.
If you now see an empty input box, you’ve succeeded!
Hello, World!
Finally, we can start writing our first programme! Go to the first cell of the Notebook, and type the following:
print(“Hello World”)
(The number at the front are line number - you don’t need to type them!)
Then click the “play” icon at the top, or press Shift + Enter. You should see something like this -
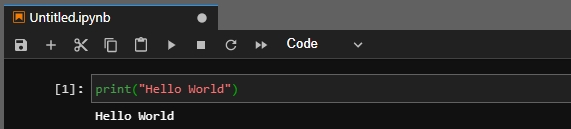
The “Hello World” Programme
Great! You have successfully installed and use Python for the first time.
Press the “save” button to save your code. And right-click the file on the left pane. You may change the name of your Jupyter notebook file.